By Paulcheeba
When using the Automated Animation (AA) module I noticed there were no hooks to bind the duration of the animations. I also noticed AA uses Sequencer to do the heavy lifting. So I went through the Sequencer Wiki on its Github and learned (pieced together) how to make a few macros for it. It is a versatile module and usable for many different applications. The main feature I am interested in is its ability to add the hook I need to link it to concentration, duration, and DAE so the hook can be automatically removed, removing the animation and effects as well.
With the following macros, I will automatically place an animated WebM video (or static webp image) asset on the scene and attach it to a token so it moves with the token. I will also set a rotating, growing intro and outro animation to the WebM video.
Originally, I animated a phasing and rotating spell effect in blender and output the video as webm so I would have this neat, animated aura effect on my token. I later learned that I can use Sequencer to, not only place and attach a video, but I can also place and attach an image instead and use Sequencer to animate it for me, adding rotation and a phasing effect IN FOUNDRY via a simple code addition to the macro. This saved me a lot of time but may have a slight impact on processing power.
Let’s get to it already!
For either method, you will create the spell item (or modify an existing one) in Foundry, give it a name and graphic, and edit the description and details tabs. Copy the SRD description from the original spell to this version, you want those sweet rollable dice buttons in the spell’s chat card details for later. The SRD versions typically apply damage on use and we don’t want that. What we want is to change the details to target self, not place a template, we don’t want saving throws and we want to use it as a utility with a duration (see notes below).
For this tutorial I assume you have the following modules installed:
- Sequencer
- Dynamic Active Effects
- Active Aura’s
- Midi-qol
- TokenMagic FX
- Times up
- JB2A Animated Spells – free version at least for their amazing Spirit Guardians animations
Optional:
- Combat Utility Belt – If you wish to add status effects to tokens in your aura range with Active Aura’s
Example Spirit Guardians Spell item settings
Dynamic Active Effects (DAE) set up
Once the spell is set up, we want to create a Dynamic Active Effect on it that will use macroExecute to run our macro that does all the coding magic. If you have Kandashi’s Active Auras installed we can even apply Active Effects to tokens that are within the aura’s range!
In the spell’s title bar click the DAE button
In the DAE WIndow click the “+” button
In this example, we have two effects: an Aura (this applies an Active Aura Effect, requiring the Active Auras Module), and an Effect (this runs the sequencer macro).
The Aura settings per tab:
- Details – Effect not suspended
- Details – Effect does not apply on equip
- Duration tab is left untouched
- Effects – Applies token magic effect to tokens within Aura range
- Auras – Specifies the Aura’s range and settings.
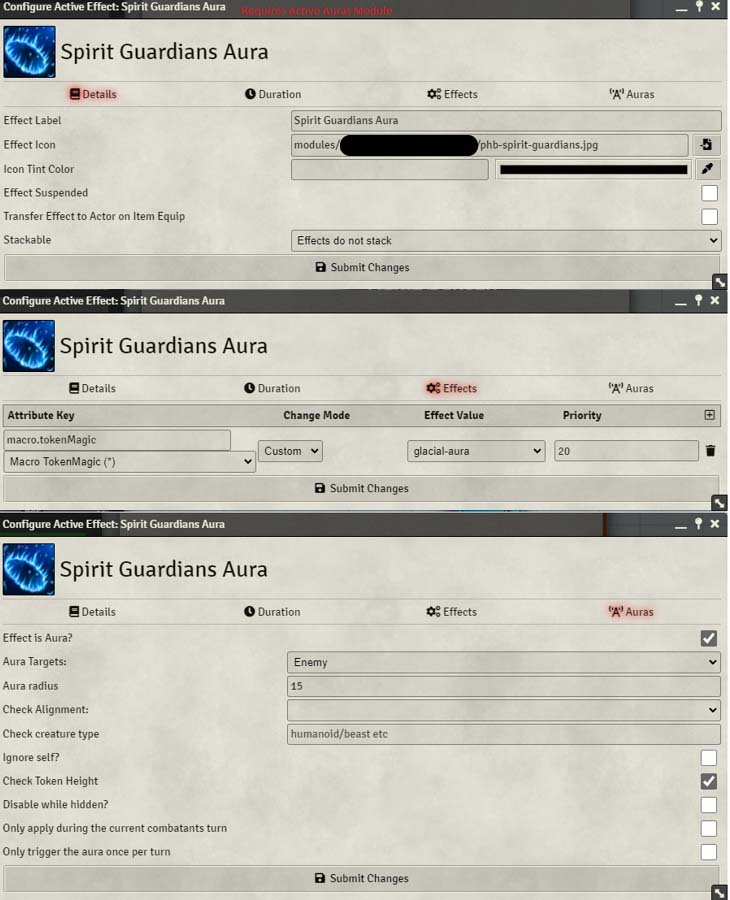
The Effect settings per tab:
- Details – Effect not suspended
- Details – Effect does not apply on equip
- Duration – Tab is left untouched
- Effects – Applies token magic effect to tokens within Aura range
- Auras – Tab is left blank.
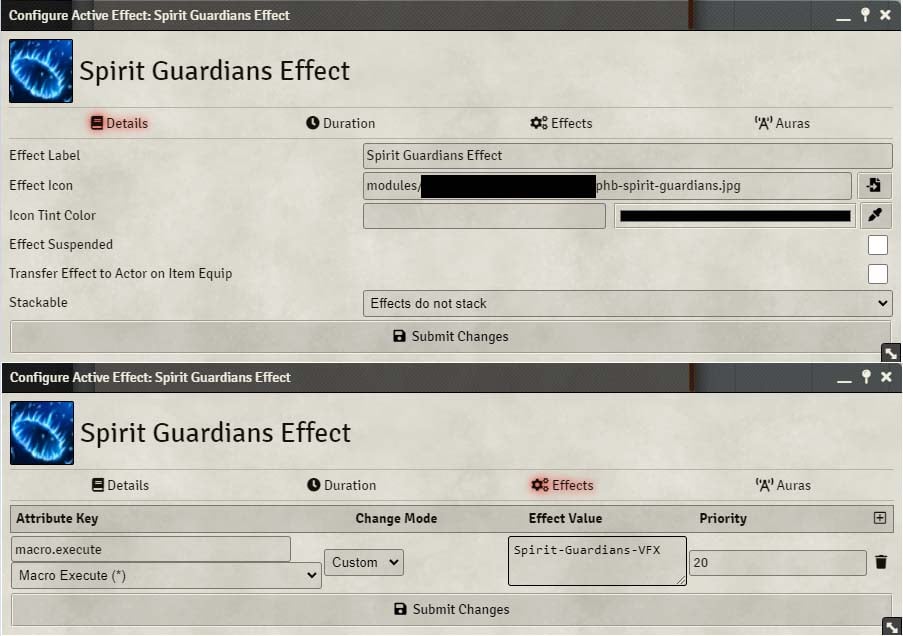
Video method, using JB2A’s Spirit Guardians animation:
In our case we want Sequencer to load the animation and apply intro and outro effects to it.
- Create a “script” macro called “Spirit-Guardians-VFX” and leave “execute as gm” unchecked.
- In the code field, paste the below code. There’s a breakdown of this code further down.
Sequencer Macro Code
let tokenD = canvas.tokens.get(args[1].tokenId);
if (args[0] === "on") {
// If the dynamic active effect started
new Sequence()
.effect()
.file("modules/jb2a_patreon/Library/3rd_Level/Spirit_Guardians/SpiritGuardians_01_Dark_Blue_600x600.webm")
.attachTo(tokenD)
.scale(1.2)
.persist()
.name(`Spirit-Guardians-${tokenD.id}`)
.fadeIn(1500, { ease: "easeOutCubic", delay: 500 })
.fadeOut(1500)
.rotateIn(90, 2500, { ease: "easeInOutCubic" })
.rotateOut(350, 1500, { ease: "easeInCubic" })
.scaleIn(1, 2500, { ease: "easeInOutCubic" })
.scaleOut(0, 1500, { ease: "easeInCubic" })
.opacity(0.7)
.belowTokens(true)
.play()
}
if (args[0] === "off") {
// If the dynamic active effect ended
Sequencer.EffectManager.endEffects({ name: `Spirit-Guardians-${tokenD.id}`, object: tokenD });
}
Code language: JavaScript (javascript)
Breakdown
let tokenD = canvas.tokens.get(args[1].tokenId); //tells sequencer to make note of the token that is casting the spell
if (args[0] === "on") { // looks to see if the DAE is applied to the token and if so tells sequencer to do something
new Sequence() //Creates a new sequence
.effect() //contains the below effects
.file("modules/jb2a_patreon/Library/3rd_Level/Spirit_Guardians/SpiritGuardians_01_Dark_Blue_600x600.webm") //Our video file
.attachTo(tokenD) //attaches it to the token sequencer made note of
.scale(1.2) //changes the scale of the repeating animation (good for if a spell effect has empty space around it)
.persist() //makes the video persistent until further notice
.name(`Spirit-Guardians-${tokenD.id}`) //gives the effect a name
.fadeIn(1500, { ease: "easeOutCubic", delay: 500 }) //intro fade in animation (duration in milliseconds, {ease: “type of easing”, add a delay: duration in ms})
.fadeOut(1500) //outro fade effect (duration in ms) we call on this later
.rotateIn(90, 2500, { ease: "easeInOutCubic" }) //intro rotate in (degrees, duration, {style})
.rotateOut(350, 1500, { ease: "easeInCubic" }) //outro rotate out (degrees, duration, {style}) we call on this later
.scaleIn(1, 2500, { ease: "easeInOutCubic" }) //intro scale in (size multiplier, duration, {style})
.scaleOut(0, 1500, { ease: "easeInCubic" }) //outro scale out (size multiplier, duration, {style}) we call on this later
.opacity(0.7) //overall opacity of effect
.belowTokens(true) //sets the animation below the token instead of above
.play() //applies the above conditions and plays the effect
}
if (args[0] === "off") { //watches for the DAE to end the tells sequencer to do something
Sequencer.EffectManager.endEffects({ name: `Spirit-Guardians-${tokenD.id}`, object: tokenD }); //ends animation, starts outro animation, completely removes Spirit Guardian Aura after outro.
}
Code language: JavaScript (javascript)
Here is an example of the end result

Static image method, using Aura of Purity:
In our case we want Sequencer to load an image and apply an intro effect, animation effect, and outro effect to it.
- Create a “script” macro called “Aura-of-Purity-VFX” and leave “execute as gm” unchecked.
- In the code field paste the below code. Then I will break it down for you.
Sequencer Macro Code
let tokenD = canvas.tokens.get(args[1].tokenId);
if (args[0] === "on") {
// If the dynamic active effect started
new Sequence()
.effect()
.file("Player%20Files/Annihilation/Animated%20Spells%20Etc/Aura-of-Purity.webp")
.attachTo(tokenD)
.scale(1.125)
.persist()
.name(`Aura-of-Purity-${tokenD.id}`)
.fadeIn(1500, { ease: "easeOutCubic", delay: 500 })
.fadeOut(1500)
.rotateIn(90, 2500, { ease: "easeInOutCubic" })
.rotateOut(350, 1500, { ease: "easeInCubic" })
.scaleIn(1, 2500, { ease: "easeInOutCubic" })
.scaleOut(0, 1500, { ease: "easeInCubic" })
.opacity(0.3)
.belowTokens(true)
.loopProperty("sprite", "rotation", { from: 0, to: -360, duration: 20000, delay: 1500 })
.filter("Glow", { color: 0x30e4ee, outerStrength: 2, innerStrength: 0 })
.play()
}
if (args[0] === "off") {
// If the dynamic active effect ended
Sequencer.EffectManager.endEffects({ name: `Aura-of-Purity-${tokenD.id}`, object: tokenD });
}
Code language: JavaScript (javascript)
Breakdown
let tokenD = canvas.tokens.get(args[1].tokenId); //tells sequencer to make note of the token that is casting the spell
if (args[0] === "on") { // looks to see if the DAE is applied to the token and if so tells sequencer to do something
new Sequence() //Creates a new sequence
.effect() //contains the below effects
.file("Player%20Files/Annihilation/Animated%20Spells%20Etc/Aura-of-Purity.webp") //Our static image file
.attachTo(tokenD) //attaches it to the token sequencer made note of
.scale(1.125) //changes the scale of the repeating animation (good for if a spell effect has empty space around it)
.persist() //makes the static image persistent until further notice
.name(Aura - of - Purity - ${ tokenD.id }) //gives the effect a name
.fadeIn(1500, { ease: "easeOutCubic", delay: 500 }) //intro fade in animation (duration in milliseconds, {ease: “type of easing”, add a delay: duration in ms})
.fadeOut(1500) //outro fade effect (duration in ms) we call on this later
.rotateIn(90, 2500, { ease: "easeInOutCubic" }) //intro rotate in (degrees, duration, {style})
.rotateOut(350, 1500, { ease: "easeInCubic" }) //outro rotate out (degrees, duration, {style}) we call on this later
.scaleIn(1, 2500, { ease: "easeInOutCubic" }) //intro scale in (size multiplier, duration, {style})
.scaleOut(0, 1500, { ease: "easeInCubic" }) //outro scale out (size multiplier, duration, {style}) we call on this later
.opacity(0.3) //overall opacity of effect
.belowTokens(true) //sets the animation below the token instead of above
.loopProperty("sprite", "rotation", { from: 0, to: -360, duration: 20000, delay: 1500 }) //slowly rotates the static image 1 full turn over 20000 ms and repeats the rotation until the effect ends
.filter("Glow", { color: 0x30e4ee, outerStrength: 2, innerStrength: 0 }) //applies a glow effect filter to make the static image a little fancier (“filter name”,{colour:red/hex code/markup code, outer effect distance, inner effect distance})
.play() //applies the above conditions and plays the effect
}
if (args[0] === "off") { //watches for the DAE to end the tells sequencer to do something
Sequencer.EffectManager.endEffects({ name: `Aura - of - Purity - ${tokenD.id}`, object: tokenD }); //ends animation, starts outro animation, completely removes Aura of Purity Aura after outro.
}
Code language: JavaScript (javascript)
Here is an example of the end result, note the immunities and resistances on the allied token
Notes
The sequence of events here is as follows:
- Cast spell on self
- Spell applies DAE on self and executes macroExecute and runs our -VFX macro and Active Aura and places a Status Marker on self
- Macro pulls image from folder, animates an intro
- Macro applies animation effect to static image or plays video on repeat
- Insert Gameplay, Roleplaying and Active Aura magic here
- Concentration or Duration ends removing DAE, Active Aura, Status Marker
- Tells the macro to animate the outro
- All traces of the spell effect are removed from the scene
All the above happens with one click of the spell.
If the spell has no Concentration or Duration, you need to remove the animation yourself by deleting the DAE that is applied to your caster.
If the spell has Duration only, you will need to wait for the duration to end or remove the animation yourself by deleting the DAE that is applied to your caster.
Of course, my file structure is different than yours, use your own file path to your video or image file.
Here is a link to the Sequencer Github Wiki for more sequencer settings and effects you can apply to your animations:
https://github.com/fantasycalendar/FoundryVTT-Sequencer/wiki
Thanks to
For creating such a killer module!
Additional thanks to the creators of the other modules used in this tutorial.
I hope this helps you learn how to use Sequencer to apply those awesome aura spells that you love as much as I do.
Cheers!
Awesome article. I love automated effects/sequencer. I don’t want Foundry to be a video game, but those extra bits of glitter are appreciated by our group.